暗黑模式
渲染管道(Rendering Pipe)
教程3DWebGL
Program
A Program 是一对函数
- Vertext Shader
- Fragment Shader
GLSL(Graphics Library Shader Language))
在 GPU 中运行
顶点着色器用于计算顶点属性,输出基元(点、线、三角形)。
然后光栅化这些基元,此时需要片元着色器来计算基元每个像素的颜色。
几乎所有 WebGL API 都是与设置这2个函数对相关的。
渲染管道
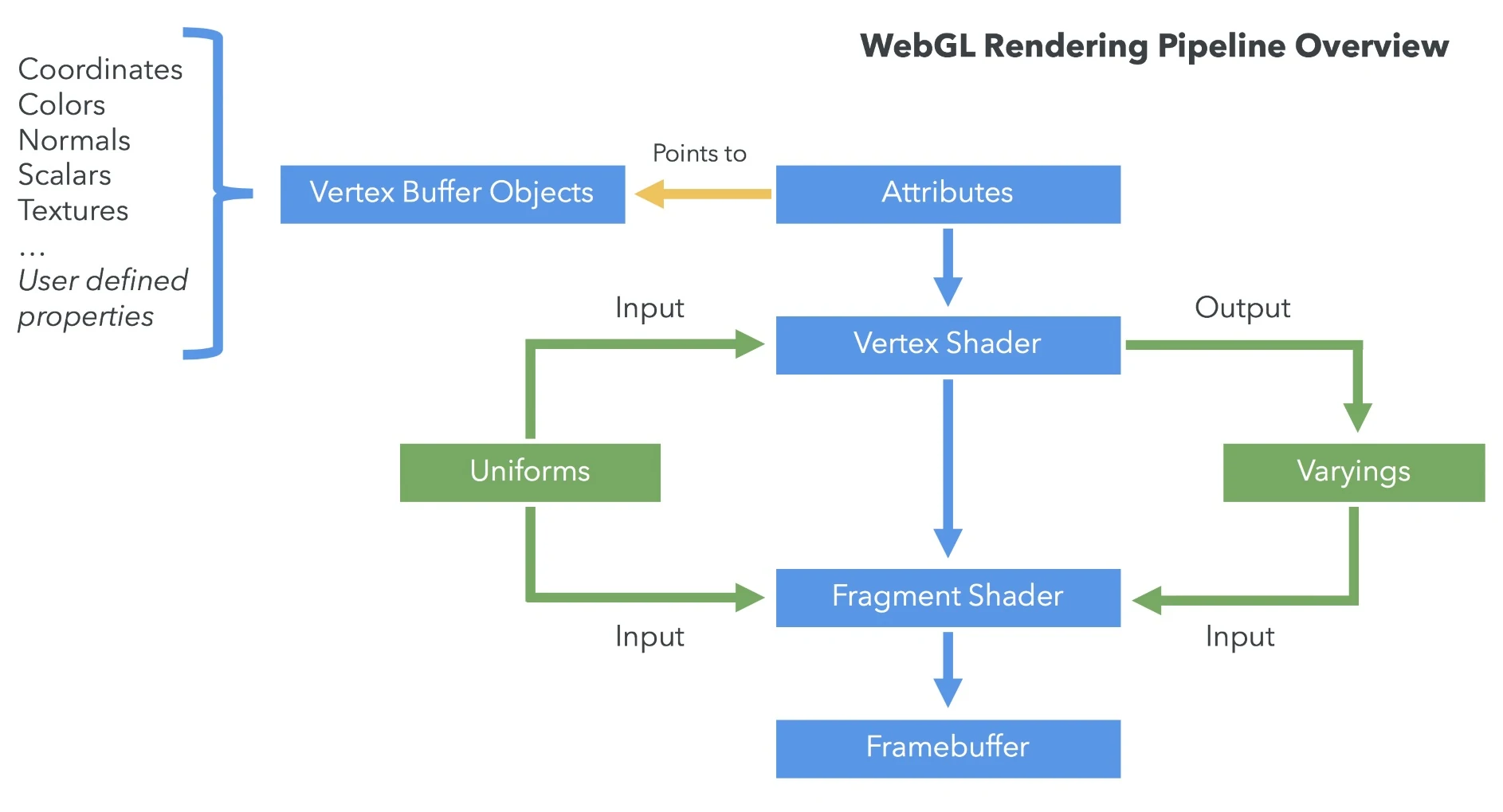
Vertex Buffer Objects (VBOs)
VBOs包含用于描述要渲染的几何图形的数据:
- 顶点坐标:定义3D对象的顶点的点
- 顶点法线
- 颜色
- 纹理坐标
Index Buffer Objects (IBOs)
IBOs描述了顶点之间的关系,用于组成模型。
IBOs使用 VBOs 的索引作为自己的值。
Vertex Shader
每个顶点都会调用顶点着色器函数。
此着色器操作每个顶点的数据,例如顶点坐标、法线、颜色和纹理坐标。 这些数据被称为 Vertex Shader 的 Attributes。
Fragment Shader
每 3 个顶点组成一个三角形,此三角形区域内的每个点都需要被赋予颜色值。每个点称为一个片元(Fragment)。 这些点映射到屏幕上,也就是像素。
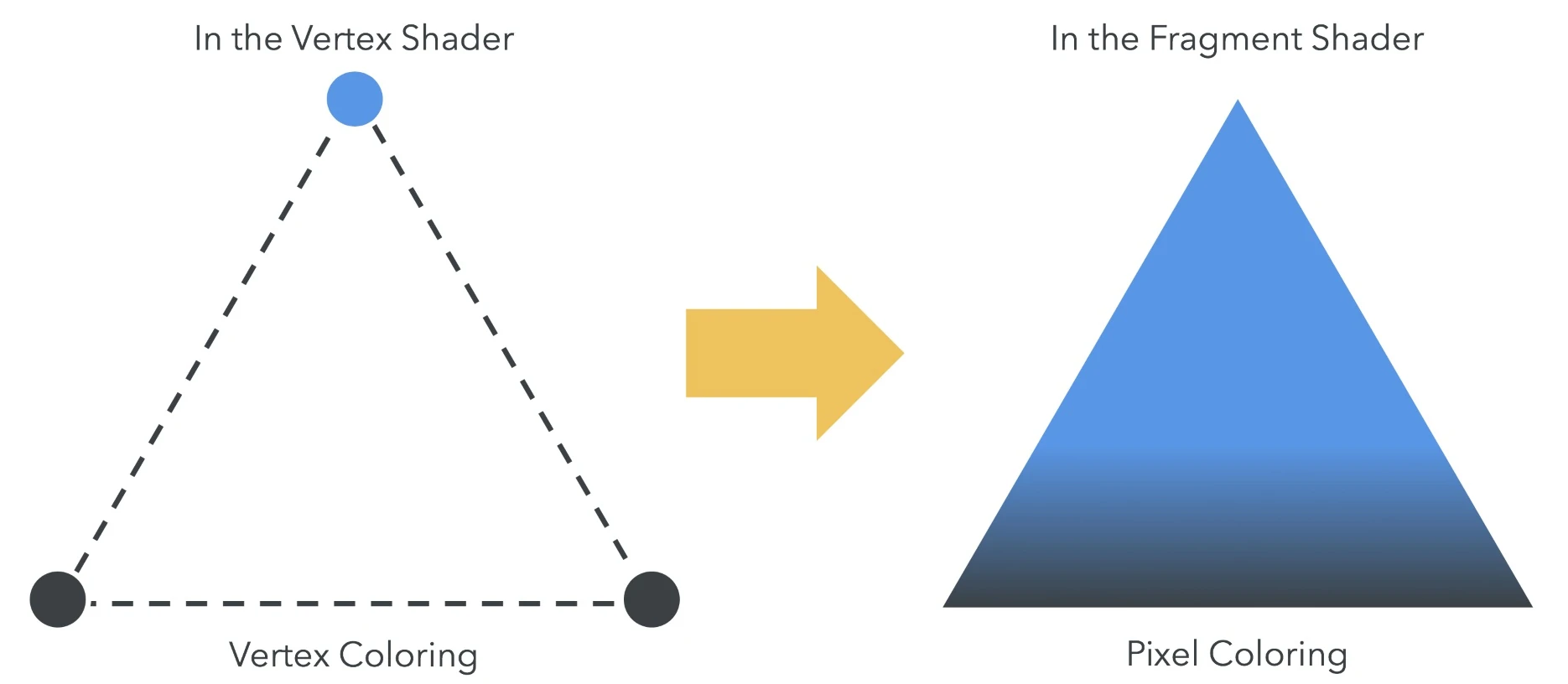
帧缓冲区(Framebuffer)
2维的缓冲区,包含了被片元着色器处理之后的片元。
当所有的片元都处理了,一张 2维 的图片就形成了并显示在屏幕上。
framebuffer 是渲染管道的最终目的地。
Attributes
Attributes 是 Vertex Shader 函数的输入变量
Attributes 决定了从 VBOs 里如何获取所需的数据。
Uniforms
Uniforms 是顶点着色器和片段着色器都可以使用的输入变量。与 Attributes 不同,Uniforms 在呈现周期中是恒定的。例如,光的位置通常被建模为 Uniforms 的。Uniforms 是你在执行着色器之前设置的全局变量。
Textures
Textures 就是数组,包含了在着色器中使用到的纹理数据。
一般就是图片数据,但也可以存放任意其他数据。
Varyings
Varyings 是用来从 Vertex Shader 向 Fragment Shader 传递数据的。
根据渲染的内容——点、线或三角形——顶点着色器在一个变量上设置的值将在执行片段着色器时被插值。
渲染
有两种数据类型是表示任何3D对象几何形状的基础:顶点 vertices 和索引 indices。
顶点
顶点是定义3D对象的角的点,用 3 个 float 类型的数值(x,y,z)表示一个顶点
- x:X 轴坐标
- y:Y 轴坐标
- z:Z 轴坐标
在 JavaScript 中用数组表示
索引 Indices
索引是给定3D场景中顶点的数字标签。索引允许我们告诉WebGL如何连接顶点以生成曲面。与顶点一样,索引存储在JavaScript数组中,然后传递给 使用WebGL索引缓冲区的WebGL渲染管道。
定义一个几何图形
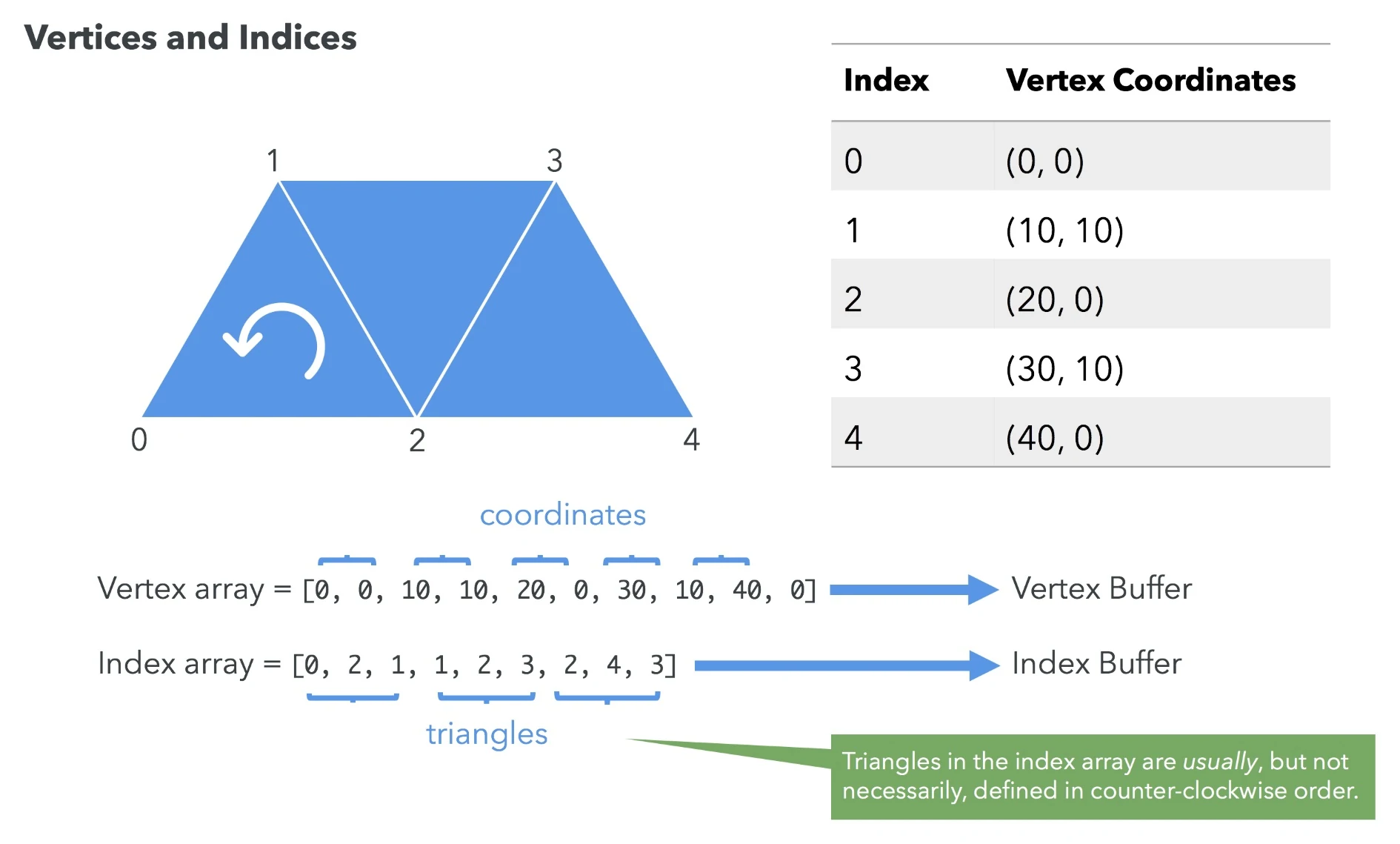
创建 buffers
关于 Typed Array
js
...
gl.bufferData(gl.ARRAY_BUFFER, new Float32Array(vertices), gl.STATIC_DRAW);
...
1
2
3
2
3
WebGL API 内部使用的数组与 JavaScript 的普通数组(Array
)是不兼容的,为了兼容并提高图形处理速度,需要把普通的 Array
转换成二进制的 TypedArray
: Int8Array
, Uint8Array
, Int16Array
, Uint16Array
, Int32Array
, Uint32Array
, Float32Array
, Float64Array
WARNING
Index Buffer: Uint16Array 严格限制
Vertex Array Objects
VAO 把顶点或索引相关的数据存储在一个对象里,方便管理和操作;
VAO 有助于性能提高
This is an important feature that should always be used, since it significantly reduces rendering times. When not using VAOs, all attributes data is in global WebGL state, which means that calling functions such as gl.vertexAttribPointer, gl.enableVertexAttribArray, and gl.bindBuffer(gl.ELEMENT_ARRAY_BUFFER, buffer) manipulates the global state. This leads to performance loss, because before any draw call, we would need to set up all vertex attributes and set the ELEMENT_ARRAY_BUFFER where indexed data is being used. On the other hand, with VAOs, we would set up all attributes during our application's initialization and simply bind the data at render, yielding much better performance.
Draw Mode
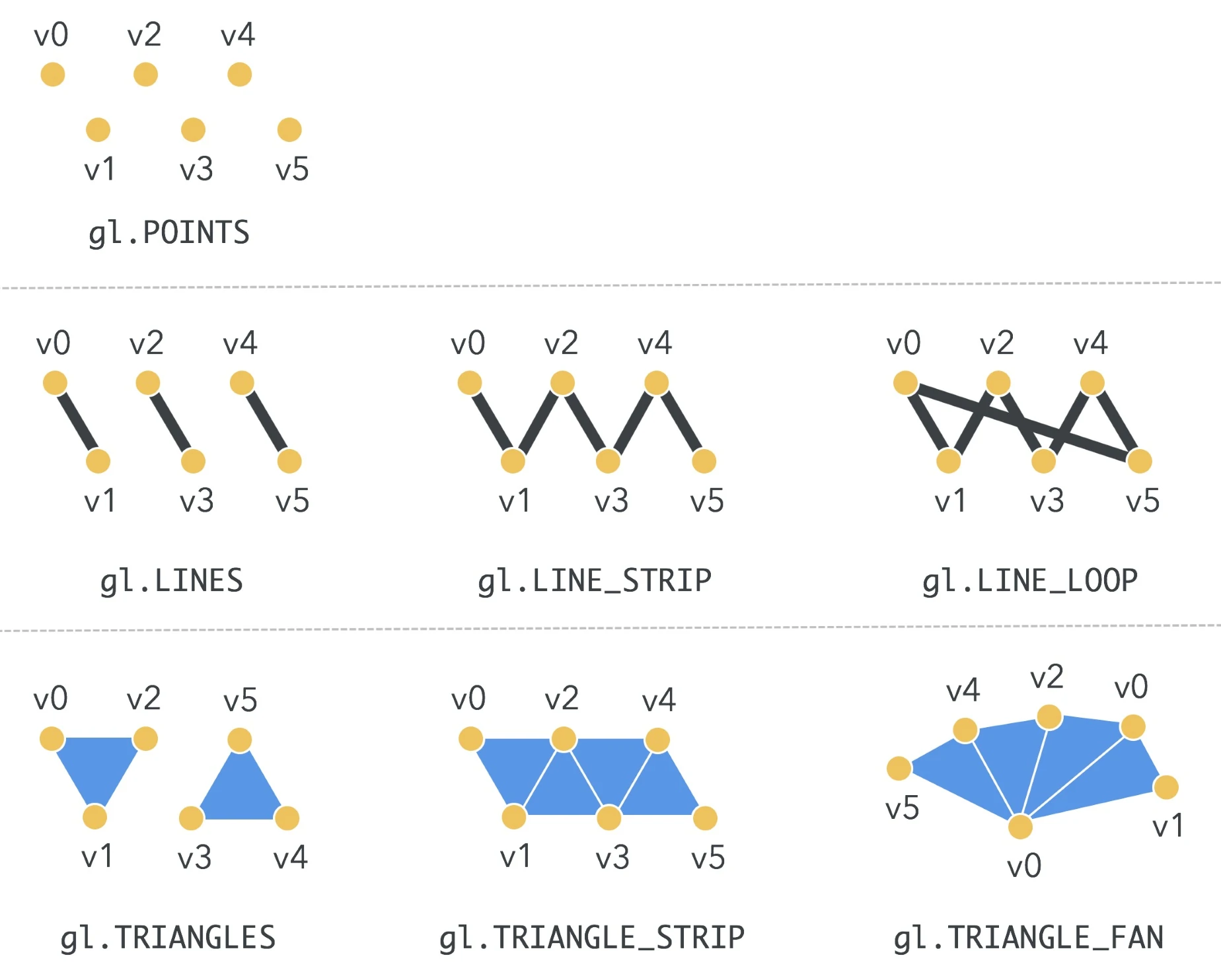
getParameter
getBufferParameter
isBuffer
getParameter(parameter)
parameter
可以是:- ARRAY_BUFFER_BINDING: Retrieves a reference to the currently-bound VBO
- ELEMENT_ARRAY_BUFFER_BINDING: Retrieves a reference to the currently-bound IBO
getBufferParameter(type, parameter)
type
可以是:- ARRAY_BUFFER: To refer to the currently-bound VBO
- ELEMENT_ARRAY_BUFFER: To refer to the currently-bound IBO
parameter
可以是:- BUFFER_SIZE: Returns the size of the requested buffer
- BUFFER_USAGE: Returns the usage of the requested buffer
isBuffer(object)
- return true if the object is a WebGL buffer, or false with an error when the buffer is invalid.
- 不需要提前绑定 VBO/VIO
AJAX 加载 JSON 模型
...